Using Markdown as a blog format
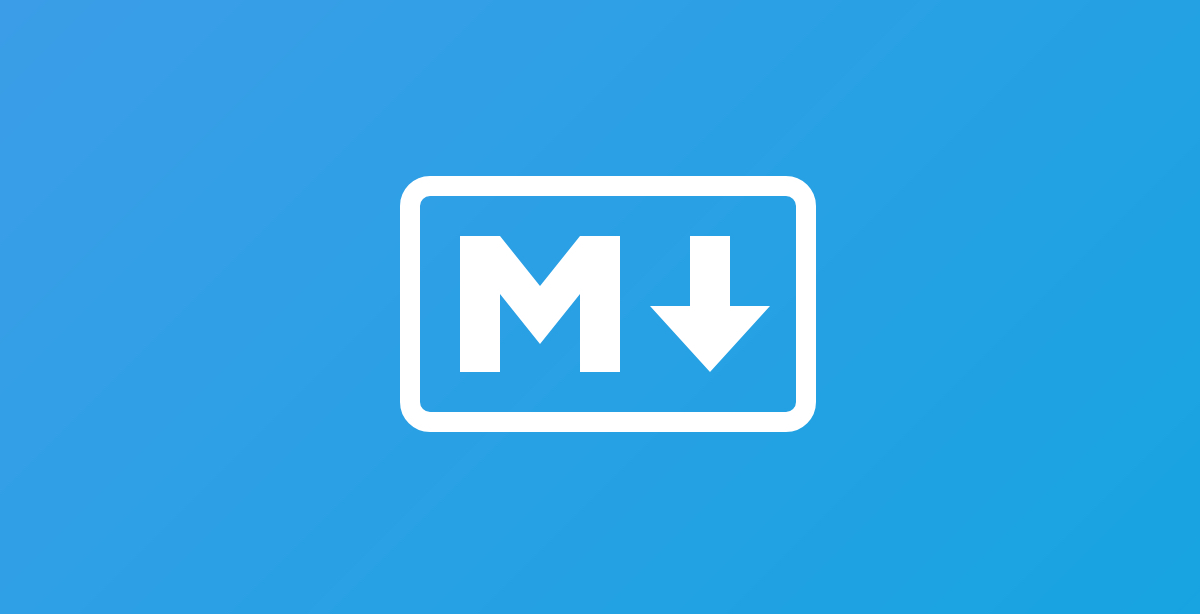
1---
2title: 'Using Markdown as a blog format'
3date: '2022-04-03'
4image: first-post.jpg
5excerpt: 'How to build a blog using react-markdown to render posts written in markdown'
6isFeatured: true
7---
8
9
1import ReactMarkdown from 'react-markdown';
2import Image from 'next/image';
3import classes from './postContent.module.scss';
4import { Prism as SyntaxHighlighter } from 'react-syntax-highlighter';
5import { atomDark } from 'react-syntax-highlighter/dist/cjs/styles/prism';
6
7const PostContent = (props) => {
8 const { post } = props;
9
10 const title = post.title;
11 const content = post.content;
12 const imagePath = `/portfolio/images/posts/${post.slug}/${post.image}`;
13
14 const customRenderers = {
15 p(paragraph) {
16 const { node } = paragraph;
17
18 if (node.children[0].tagName === 'img') {
19 const image = node.children[0];
20
21 return (
22 <div>
23 <Image src={imagePath} alt={image.alt} width={450} height={450} />
24 </div>
25 );
26 }
27
28 return <p>{paragraph.children}</p>;
29 },
30
31 code(code) {
32 const { className, children } = code;
33 const language = className.split('-')[1]; // className is something like language-js => We need the "js" part here
34
35 return (
36 <SyntaxHighlighter
37 language={language}
38 style={atomDark}
39 // eslint-disable-next-line react/no-children-prop
40 children={children}
41 />
42 );
43 },
44 };
45
46 return (
47 <div className={classes.postContent}>
48 <div className={classes.container}>
49 <article>
50 <ReactMarkdown components={customRenderers}>{content}</ReactMarkdown>
51 </article>
52 </div>
53 </div>
54 );
55};
56
57export default PostContent;
58